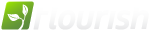
In a previous suggestion I talked about a widget system that I'm working with (this thread can be found here, http://flourishlib.com/discussion/2/261)
As this system has gotten some more real world use it has become necessary, for my project, to have a way to place an element at the beginning of an fTemplating array.
Using some of the code from before:
//In the common init.php
$template = new fTemplating('/path/to/templates/');
//Get a handle to the special widget, it will be shown at the top of a special page
$template->set('special-widget', 'widgets/special.widget.php');
//On most pages we will show the a, b, c, and d widgets
$template->set('a-widget', 'widgets/a.widget.php');
$template->set('b-widget', 'widgets/b.widget.php');
$template->set('c-widget', 'widgets/c.widget.php');
$template->set('d-widget', 'widgets/d.widget.php');
$template->add('widgets', 'a-widget');
$template->add('widgets', 'b-widget');
$template->add('widgets', 'c-widget');
$template->add('widgets', 'd-widget');
Then later on being able to prepend allows me to do something like this
//Put the special widget first
$template->prepend('widgets', 'special-widget');
$template->place('widget-panel');
To acheive this I added a method to the fTemplating class following the same code style as the rest of the class.
/**
* Adds a value to the beginning of an array element
*
* @param string $element The element to add to
* @param mixed $value The value to add
* @param fTemplating The template object to allow for method chaining
*/
public function prepend($element, $value) {
if(!isset($this->elements[$element])) {
$this->elements[$element] = array();
}
if(!is_array($this->elements[$element])) {
throw new fProgrammerException(
'%1$s was called for an element, %2$s, which is not an array',
'prepend()',
$element
);
}
array_unshift($this->elements[$element], $value);
return $this;
}
For the sake of symmetry I added an append function that simply calls the add function.
This was useful for my use-case but may not be generally useful. I submit this code for consideration of inclusion.