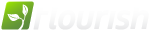
In using the fTemplating class I've found the fTemplating::add() method to be a handy way to encapsulate arrays of display elements that can change from page to page. My use case has a fairly common default, so I set that up in a common init script. I decided that it would be helpful to have a symmetric fTemplating::remove() function to remove a value from an array. An example is provided below.
//In the common init.php
$template = new fTemplating('/path/to/templates/');
//On most pages we will show the a, b, c, and d widgets
$template->set('a-widget', 'widgets/a.widget.php');
$template->set('b-widget', 'widgets/b.widget.php');
$template->set('c-widget', 'widgets/c.widget.php');
$template->set('d-widget', 'widgets/d.widget.php');
$template->add('widgets', 'a-widget');
$template->add('widgets', 'b-widget');
$template->add('widgets', 'c-widget');
$template->add('widgets', 'd-widget');
Then later on in some special page that doesn't show the c-widget for some reason
//Special page, don't show the c-widget
$template->remove('widgets', 'c-widget');
$template->place('widget-panel');
To acheive this I added a method to the fTemplating class following the same code style as the rest of the class.
/**
* Removes a value from an array element
*
* @param string $element The element to remove from
* @param mixed $value The value to remove
* @return fTemplating The template object, to allow for method chaining
*/
public function remove($element, $value) {
if(!is_array($this->elements[$element])) {
throw new fProgrammerException(
'%1$s was called for an element, %2$s, which is not an array',
'remove()',
$element
);
}
$key = array_search($value, $this->elements[$element]);
if($key === FALSE) {
throw new fProgrammerException(
'%1$s was called for a value, %2$s, which is not in element, %3$s',
'remove()',
$value,
$element
);
} else {
unset($this->elements[$element][$key]);
}
return $this;
}
I would like to release this code to be considered for inclusion in the Flourish library.