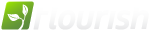
Creating a very basic user table and attempting to check for a user upon login. This file uses namespaces so global
is used.
DROP TABLE IF EXISTS users;
CREATE TABLE users (
uid INTEGER PRIMARY KEY AUTO_INCREMENT,
name VARCHAR(64) NOT NULL,
pass VARCHAR(64) NOT NULL,
access INTEGER UNSIGNED NOT NULL DEFAULT 0,
created INTEGER UNSIGNED NOT NULL DEFAULT 0
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE UNIQUE INDEX uid_name ON users (uid, name);
public function validate() {
try {
$hasher = new \\PasswordHash(8, TRUE);
$hash = $hasher->HashPassword($this->post['pass']);
$user = new \\Base\\User(array('name' => $this->post['name'], 'pass' => $hash));
}
catch (\\fNotFoundException $e) {
die($e->getMessage());
}
}
I get Fatal error: Uncaught exception 'fProgrammerException' with message 'An invalidly formatted primary or unique key was passed to this User object'