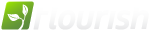
I've got a problem where I need to "escape" user input for a part of a query that is NOT a value. Here's the scenario:
I'm using jqGrid to display many tables with dozens of different column names. If a user sorts or filters and column, I get the request via AJAX. I then know what columns to order on.
I've developed one master query that will query the appropriate table, the right columns, group, order by, limit etc. This way, I don't need a query for each table.
However, the problem is with the risk of SQL injection on the order by or group by clause as the column names are provided by the user. I searched a bit and found a few discussions on this, but they all seem to say the same thing : use white lists of column names to compare against. That is probably my only solution, but it is not very friendly to future changes.
Do you have any suggestions for this within flourish? Of course, you can't escape these because that would put quotes around them and produce invalid SQL. Ideas?
FYI : http://josephkeeler.com/2009/05/php-security-sql-injection-in-order-by/
Also, I am using raw SQL queries. I can imagine that fORM could prevent this as it knows what all columns are actually in the db. However, I've got some pretty complex queries that are easiest to do directly with SQL.