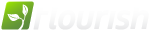
Are you thinking of creating a branch of flourish, that would implement name spacing and use some of the other advantages of PHP 5.3.
Below is a extension of the fCryptography class to provide more secure passwords through the crypt(which uses cryptology functions only availably natively in php 5.3) function.
namespace Flourish\\Utilities;
use \\fCryptography;
class Cryptography{
static public function hashPassword($password)
{
if(function_exists('crypt') && CRYPT_SHA512 == 1){
$salt = fCryptography::randomString(16);
}else if(function_exists('crypt') && CRYPT_SHA256 == 1){
$salt = fCryptography::randomString(16);
}else if(function_exists('crypt') && CRYPT_BLOWFISH == 1){
$salt = fCryptography::randomString(22,'base64');
}else{
$salt = fCryptography::randomString(10);
}
return self::hashWithSalt($password,$salt);
}
static public function checkPasswordHash($password, $hash){
$hash_parts = explode('$',$hash);
array_shift($hash_parts);
if(count($hash_parts) > 1){
$salt = $hash_parts[2];
}else{
$salt = substr($hash, 29, 10);
}
return self::hashWithSalt($password, $salt) === $hash;
}
static private function hashWithSalt($source, $salt){
if(function_exists('crypt') && CRYPT_SHA512 == 1){
$hash = crypt($source, '$6$rounds=5000$'.$salt.'$');
}else if(function_exists('crypt') && CRYPT_SHA256 == 1){
$hash = crypt($source, '$5$rounds=5000'.fCryptography::randomString(16).'$');
}else if(function_exists('crypt') && CRYPT_BLOWFISH == 1){
$hash = crypt($source, '$2a$14$'.fCryptography::randomString(22,'base64').'$');
}else{
$sha1 = sha1($salt . $source);
for ($i = 0; $i < 1000; $i++) {
$sha1 = sha1($sha1 . (($i % 2 == 0) ? $source : $salt));
}
$hash = 'fCryptography::password_hash#' . $salt . '#' . $sha1;
}
return $hash;
}
}