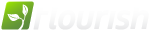
I'm trying out flourish, so far I love it, but I'm running into some difficulty with fRecordSet. My database is four tables in postgresql, full RI with primary and foreign keys, etc. The tables are:
There are other fields, but those are the ones related to the relations.
fActiveRecord based classes exist for each table and are named appropriately, e.g.
class city extends fActiveRecord
{
protected function configure()
{
}
}
Given cities.name and state.short_name, I'm trying to find the locations that are in that city and state. The raw SQL would be:
SELECT *
FROM locations lo, zipcodes zi, cities ci, states st
WHERE lo.zipcode_id = zi.zipcode_id
AND zi.city_id = ci.city_id
AND ci.state_id = st.state_id
AND ci.name = 'Dover'
AND st.short_name = 'NH'
This raw SQL works as expected.
I'm having a bit of trouble coming up with the most efficient way to retrieve this data using the fActiveRecord classes (which are defined for all the tables), and/or fRecordSet class. Rather than go over all the obviously wrong things I've tried, can someone suggest what they would do in order to get this result?