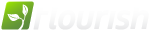
I have a database schema with two tables, 'users' and 'groups', and a joining table, 'user_groups'. The users and groups tables are very straightforward (I put only relevant fields, for briefness):
CREATE TABLE users (
id serial PRIMARY KEY,
uid varchar(32),
name varchar(64),
surname varchar(64),
);
CREATE TABLE groups (
id serial PRIMARY KEY,
groupid varchar(16) DEFAULT '',
groupname varchar(128) DEFAULT '',
active boolean DEFAULT FALSE,
);
CREATE TABLE users_groups (
id_user integer NOT NULL REFERENCES users(id) ON DELETE CASCADE,
id_group integer NOT NULL REFERENCES groups(id) ON DELETE CASCADE,
PRIMARY KEY (id_user, id_group)
);
This schema looks fine and fCore::expose($schema->getRelationships('users')) shows the following for users table:
Array
(
[one-to-one] => Array
(
)
[many-to-one] => Array
(
)
[one-to-many] => Array
(
)
[many-to-many] => Array
(
[0] => Array
(
[table] => users
[column] => id
[related_table] => groups
[related_column] => id
[join_table] => users_groups
[join_column] => id_user
[join_related_column] => id_group
[on_update] => no_action
[on_delete] => cascade
)
)
)
However, so long as each user could have a distinct role in each group he is member of, I would need to add a 'role' field to the user_groups table:
CREATE TABLE users_groups (
id_user integer NOT NULL REFERENCES users(id) ON DELETE CASCADE,
id_group integer NOT NULL REFERENCES groups(id) ON DELETE CASCADE,
role varchar(16) DEFAULT '',
PRIMARY KEY (id_user, id_group)
);
The problem is that after adding that new field, the many-to-many relationship is lost:
Array
(
[one-to-one] => Array
(
)
[many-to-one] => Array
(
)
[one-to-many] => Array
(
[0] => Array
(
[table] => users
[column] => id
[related_table] => users_groups
[related_column] => id_user
[on_delete] => cascade
[on_update] => no_action
)
)
[many-to-many] => Array
(
)
)
I don't see the reason for which it doesn't work, so long as the foreign keys are the same ones and they are single column FOREIGN KEY constraints. Any help?
A.