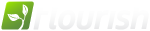
Handles filesystem-level tasks including filesystem transactions and the reference map to keep all fFile and fDirectory objects in sync
1.0.0b16 | Added a call to clearstatcache() to rollback() to prevent incorrect data from being returned by fFilegetMTime() and fFile::getSize() 11/27/10 |
---|---|
1.0.0b15 | Fixed translateToWebPath() to handle Windows \s 4/9/10 |
1.0.0b14 | Added recordAppend() 3/15/10 |
1.0.0b13 | Changed the way files/directories deleted in a filesystem transaction are handled, including improvements to the exception that is thrown 3/5/10 |
1.0.0b12 | Updated convertToBytes() to properly handle integers without a suffix and sizes with fractions 11/14/09 |
1.0.0b11 | Corrected the API documentation for getPathInfo() 9/9/09 |
1.0.0b10 | Updated updateExceptionMap() to not contain the Exception class parameter hint, allowing NULL to be passed 8/20/09 |
1.0.0b9 | Added some performance tweaks to createObject() 8/6/09 |
1.0.0b8 | Changed formatFilesize() to not use decimal places for bytes, add a space before and drop the B in suffixes 7/12/09 |
1.0.0b7 | Fixed formatFilesize() to work when $bytes equals zero 7/8/09 |
1.0.0b6 | Changed replacement values in preg_replace() calls to be properly escaped 6/11/09 |
1.0.0b5 | Changed formatFilesize() to use proper uppercase letters instead of lowercase 6/2/09 |
1.0.0b4 | Added the createObject() method 1/21/09 |
1.0.0b3 | Removed some unnecessary error suppresion operators 12/11/08 |
1.0.0b2 | Fixed a bug where the filepath and exception maps weren't being updated after a rollback 12/11/08 |
1.0.0b | The initial implementation 3/24/08 |
Adds a directory to the web path translation list
The web path conversion list is a list of directory paths that will be converted (from the beginning of filesystem paths) when preparing a path for output into HTML.
By default the $_SERVER['DOCUMENT_ROOT'] will be converted to a blank string, in essence stripping it from filesystem paths.
void addWebPathTranslation( string $search_path, string $replace_path )
string | $search_path | The path to look for |
string | $replace_path | The path to replace with |
Starts a filesystem pseudo-transaction, should only be called when no transaction is in progress.
Flourish filesystem transactions are NOT full ACID-compliant transactions, but rather more of an filesystem undo buffer which can return the filesystem to the state when begin() was called. If your PHP script dies in the middle of an operation this functionality will do nothing for you and all operations will be retained, except for deletes which only occur once the transaction is committed.
void begin( )
Commits a filesystem transaction, should only be called when a transaction is in progress
void commit( )
Takes a file size including a unit of measure (i.e. kb, GB, M) and converts it to bytes
Sizes are interpreted using base 2, not base 10. Sizes above 2GB may not be accurately represented on 32 bit operating systems.
integer convertToBytes( string $size )
string | $size | The size to convert to bytes |
The number of bytes represented by the size
Takes a filesystem path and creates either an fDirectory, fFile or fImage object from it
fDirectory|fFile|fImage createObject( string $path )
string | $path | The path to the filesystem object |
Takes the size of a file in bytes and returns a friendly size in B/K/M/G/T
string formatFilesize( integer $bytes, integer $decimal_places=1 )
integer | $bytes | The size of the file in bytes |
integer | $decimal_places | The number of decimal places to display |
Returns info about a path including dirname, basename, extension and filename
array getPathInfo( string $path, string $element=NULL )
string | $path | The file/directory path to retrieve information about |
string | $element | The piece of information to return: 'dirname', 'basename', 'extension', or 'filename' |
The file's dirname, basename, extension and filename
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Hooks a file/directory into the deleted backtrace map entry for that filename
Since the value is returned by reference, all objects that represent this file/directory always see the same backtrace.
mixed &hookDeletedMap( string $file )
string | $file | The name of the file or directory |
Will return NULL if no match, or the backtrace array if a match occurs
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Hooks a file/directory name to the filename map
Since the value is returned by reference, all objects that represent this file/directory will always be update on a rename.
mixed &hookFilenameMap( string $file )
string | $file | The name of the file or directory |
Will return NULL if no match, or the exception object if a match occurs
Indicates if a transaction is in progress
void isInsideTransaction( )
Returns a unique name for a file
string makeUniqueName( string $file, string $new_extension=NULL )
string | $file | The filename to check |
string | $new_extension | The new extension for the filename, should not include . |
The unique file name
Changes a filename to be safe for URLs by making it all lower case and changing everything but letters, numers, - and . to _
string makeURLSafe( string $filename )
string | $filename | The filename to clean up |
The cleaned up filename
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Stores what data has been added to a file so it can be removed if there is a rollback
void recordAppend( fFile $file, string $data )
fFile | $file | The file that is being written to |
string | $data | The data being appended to the file |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Keeps a record of created files so they can be deleted up in case of a rollback
void recordCreate( object $object )
object | $object | The new file or directory to get rid of on rollback |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Keeps track of file and directory names to delete when a transaction is committed
void recordDelete( fFile|fDirectory $object )
fFile|fDirectory | $object | The filesystem object to delete |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Keeps a record of duplicated files so they can be cleaned up in case of a rollback
void recordDuplicate( fFile $file )
fFile | $file | The duplicate file to get rid of on rollback |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Keeps a temp file in place of the old filename so the file can be restored during a rollback
void recordRename( string $old_name, string $new_name )
string | $old_name | The old file or directory name |
string | $new_name | The new file or directory name |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Keeps backup copies of files so they can be restored if there is a rollback
void recordWrite( fFile $file )
fFile | $file | The file that is being written to |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Resets the configuration of the class
void reset( )
Rolls back a filesystem transaction, it is safe to rollback when no transaction is in progress
void rollback( )
Takes a filesystem path and translates it to a web path using the rules added
string translateToWebPath( string $path )
string | $path | The path to translate |
The filesystem path translated to a web path
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Updates the deleted backtrace for a file or directory
void updateDeletedMap( string $file, array $backtrace )
string | $file | A file or directory name, directories should end in / or \ |
array | $backtrace | The backtrace for this file/directory |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Updates the filename map, causing all objects representing a file/directory to be updated
void updateFilenameMap( string $existing_filename, string $new_filename )
string | $existing_filename | The existing filename |
string | $new_filename | The new filename |
Please note: this method is public, however it is primarily intended for internal use by Flourish and will normally not be useful in site/application code
Updates the filename map recursively, causing all objects representing a directory to be updated
Also updates all files and directories in the specified directory to the new paths.
void updateFilenameMapForDirectory( string $existing_dirname, string $new_dirname )
string | $existing_dirname | The existing directory name |
string | $new_dirname | The new dirname |